Hero Jump 예제
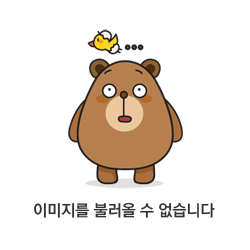
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.Experimental.XR.Interaction;
public class HeroController : MonoBehaviour
{
Animator anim;
private Rigidbody2D rBody2D;
public float moveForce = 10f;
public float moveSpeed = 1f;
private bool isJump = false;
float h;
float jumpForce = 1f;
//State: 0:idle,1:run,2:jump
// Start is called before the first frame update
void Start()
{
this.anim = GetComponent<Animator>();
this.rBody2D = GetComponent<Rigidbody2D>();
}
// Update is called once per frame
void Update()
{
h = Input.GetAxisRaw("Horizontal"); //-1, 0, 1
// Debug.LogFormat("=> {0}", (int)h);
// Vector2 vector = new Vector2(0, 0);
if (Input.GetKeyDown(KeyCode.Space))
{//스페이스바 누르면 점프)
{
// Debug.LogError("Jump");
Debug.Log("Jump");
//rBody2D.velocity = Vector2.up * jumpForce;
this.rBody2D.AddForce(Vector2.up * this.jumpForce, ForceMode2D.Impulse);
// Debug.LogFormat("after jump velocity:{0}", rBody2D.velocity);
if (this.isJump == false)
{
this.isJump = true;
}
}
}
if (h != 0)//이동중
{
if (!this.isJump)
this.anim.SetInteger("State", 1);
this.transform.localScale = new Vector3(h, 1, 1);
Vector2 force = Vector2.zero;
if (this.isJump)//점프하면서 이동
{
force = new Vector2(h, 0) * this.moveForce * 0.5f;
if (Mathf.Abs(force.x) > 15f)
force.x = 15f * h;
this.rBody2D.AddForce(force);
}
else //점프안하고 이동
{
force = new Vector2(h, 0) * this.moveForce;
if (Mathf.Abs(force.x) > 15f)
force.x = 15f * h;
this.rBody2D.AddForce(force);
}
}
else //멈춤: h == 0
{
// Debug.Log(h);
if (!this.isJump)
this.anim.SetInteger("State", 0);
else
{
this.anim.SetInteger("State", -1);//점프하고 멈춤
if (this.rBody2D.velocity.y > 0)
{
this.anim.SetInteger("State", 2);//Jump
}
else if (this.rBody2D.velocity.y < 0)
{
// Debug.LogFormat("{0}", this.rBody2D.velocity.y);
this.anim.SetInteger("State", 3);//JumpDown
}
}
}
}
//private void OnCollisionEnter2D(Collision2D collision)
//{
// if (collision.gameObject.tag == "Ground")
// {
// if (this.isJump)
// {
// this.isJump = false;
// Debug.LogFormat("h ===> {0}", this.h);
// if (this.h != 0)
// {
// //run
// Debug.Log("Run!");
// this.anim.SetInteger("State", 1);
// }
// else
// {
// //idle
// Debug.Log("Idle");
// this.anim.SetInteger("State", 0);
// }
// }
// }
//}
}
'유니티 기초' 카테고리의 다른 글
Unity- 버튼 클릭(Onclick)하면 이동 (0) | 2023.08.04 |
---|---|
unity - 3d 기본 예제-밤송이 (0) | 2023.08.04 |
rigidbody가 없을 때 이동: Translate (0) | 2023.08.03 |
애니메이션 전환 연습 (0) | 2023.08.03 |
Unity-Physics,Rigidbody, 스프라이트 애니메이션, ClimbCloud 예제 (0) | 2023.08.02 |